Redis is an open-source, in-memory data structure store that can be used as a database, cache, and message broker. It is known for its fast performance, scalability, and flexibility. Redis supports various data structures like strings, hashes, lists, sets, and sorted sets, and it also provides powerful features like pub/sub messaging, transactions, and Lua scripting.
Practical Example:
Let’s say you are building a web application that needs to store session data for logged-in users. You could use Redis to store this data as it provides fast and efficient key-value storage.
To get started, you would first need to install Redis on your machine or server. Once installed, you can start a Redis server by running the following command:
redis-server
Or, if you already have redis-server installed and running you can check it ( and get the running port ) using:
sudo systemctl status redis
Now, let’s write some code to interact with Redis. Here’s an example in Python using the Redis-py library:
import redis
# connect to Redis
r = redis.Redis(host='localhost', port=6379, db=0)
# set a value
r.set('username', 'johndoe')
# get a value
username = r.get('username')
print(username.decode('utf-8'))
In this example, we connect to Redis using the Redis-py library and set a value for the key ‘username’. We then retrieve the value for that key and print it out.
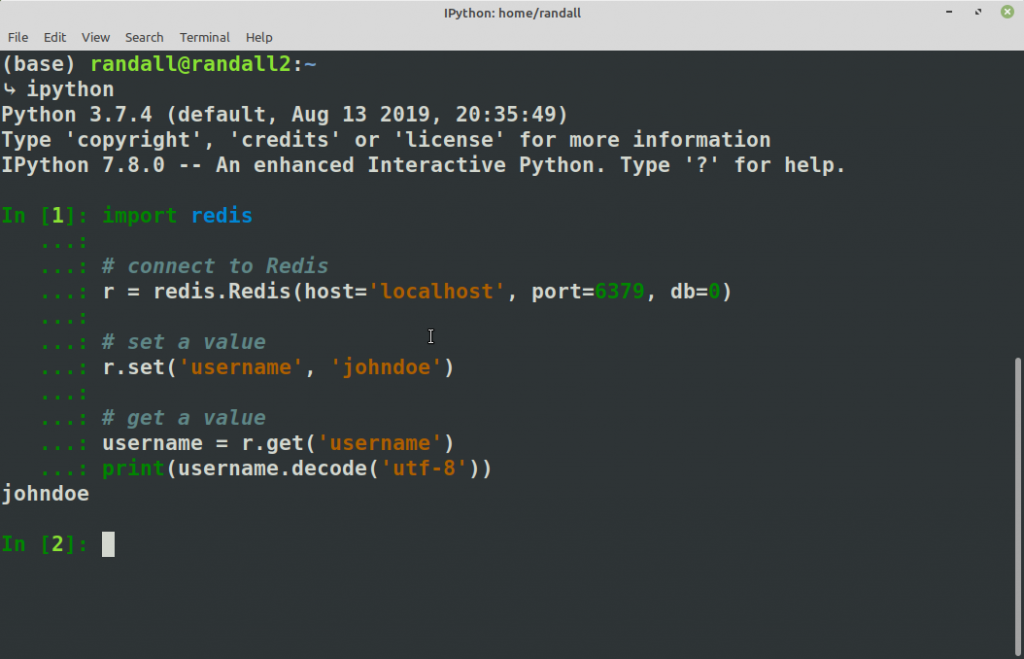
This is just a simple example, but Redis can be used for much more complex tasks like caching, message queuing, and real-time analytics. With its ease of use and powerful features, Redis is a great tool to have in your toolbox.
Directly interact with Redis using the redis-cli
The remaining examples can be run directly against the redis server using the CLI. Even thou
To start the Redis CLI, open a terminal or shell and enter the following command:
redis-cli
This will open the Redis CLI and connect to a Redis server running on the default host (localhost
) and port (6379
). If your Redis server is running on a different host or port, you can specify it using the -h
and -p
options:
redis-cli -h <hostname> -p <port>
Once you are connected to the Redis server, you can start executing Redis commands. For example, to set a key-value pair, you can use the SET
command:
127.0.0.1:6379> SET mykey "Hello World"
OK
To retrieve the value of a key, you can use the GET
command:
127.0.0.1:6379> GET mykey
"Hello World"
You can also exit the Redis CLI by typing exit
or quit
.
Note that the Redis CLI is a powerful tool for interacting with Redis, but it may not be the most suitable tool for all use cases. There are many Redis client libraries available for various programming languages that provide more advanced functionality and are better suited for application development.
Modify the Value in a key,value pair.
There a few ways you can modify the value in a key,value pair in situ.
SET key value [EX seconds] [PX milliseconds] [NX|XX]
:
Sets the value of a key. If the key already exists, the value is overwritten. If theXX
option is used, the command only succeeds if the key already exists. If theNX
option is used, the command only succeeds if the key does not already exist. TheEX
andPX
options allow you to set an expiration time for the key.SETRANGE key offset value
:
Sets a substring of the value of a key starting at the specified offset.APPEND key value
:
Appends the specified value to the existing value of a key.INCR key
:
Increments the value of a key by 1.INCRBY key increment
:
Increments the value of a key by the specified increment.DECR key
:
Decrements the value of a key by 1.DECRBY key decrement
:
Decrements the value of a key by the specified decrement.
Here are some examples of setting key-value pairs on Redis using the CLI:
- Setting a string value:
127.0.0.1:6379> SET name "John"
OK
- Setting a numeric value:
127.0.0.1:6379> SET age 35
OK
- Setting multiple key-value pairs at once:
127.0.0.1:6379> MSET city "New York" occupation "Engineer"
OK
- Setting a value with an expiration time (in seconds):
127.0.0.1:6379> SET token "mysecrettoken" EX 3600
OK
In this example, the token
key is set with a value of "mysecrettoken"
and an expiration time of 3600 seconds (1 hour).
- Setting a value only if the key does not already exist:
127.0.0.1:6379> SETNX username "johndoe"
(integer) 1
In this example, the SETNX
command sets the username
key to "johndoe"
only if the key does not already exist.
These are just a few examples of setting key-value pairs on Redis using the CLI. You can explore more Redis commands and options using the redis-cli --help
command or by consulting the Redis documentation.
Deleting a Key,Value pair
To delete a value in Redis, you can use the DEL
command, which removes the specified key and its associated value(s) from the database. The syntax of the DEL
command is as follows:
DEL key [key ...]
Where key
is the name of the key(s) you want to delete.
Here’s an example of how to delete a key and its value using the DEL
command:
> SET mykey "Hello World"
OK
> DEL mykey
(integer) 1
> GET mykey
(nil)
In this example, we first set the value of the key mykey
to "Hello World"
. We then use the DEL
command to delete the key and its value from the database. Finally, when we attempt to retrieve the value of mykey
using the GET
command, we receive a nil
response, indicating that the key no longer exists in the database.
It’s important to note that deleting a key is a permanent operation and cannot be undone. Also, be cautious when using the DEL
command in production environments to avoid deleting important data accidentally.
Here are 10 more common Redis use cases:
- Caching: Redis can be used as a caching layer to store frequently accessed data in memory, reducing the need to make expensive database calls.
- Session Store: Redis is commonly used to store session data for web applications, allowing users to remain logged in and maintain their session state.
- Pub/Sub Messaging: Redis supports publish/subscribe messaging, allowing different parts of a system to communicate with each other in real-time.
- Real-time Analytics: Redis can be used to store and analyze real-time data, making it a powerful tool for real-time analytics and monitoring.
- Leaderboards and Rankings: Redis’s sorted set data structure can be used to create leaderboards and rankings in applications.
- Task Queues: Redis can be used to implement task queues, allowing for background processing of jobs in a scalable and efficient manner.
- Geo-spatial Indexing: Redis supports geo-spatial indexing, allowing you to store and query geo-location data.
- Rate Limiting: Redis can be used to implement rate limiting, preventing excessive usage of resources in a system.
- Data Structures: Redis provides a variety of data structures like lists, sets, and hashes, allowing for efficient storage and retrieval of structured data.
- Full-text Search: Redis supports full-text search using the Redisearch module, making it a useful tool for applications that require advanced search functionality.